Sharing Python Code as Modules Between ROS Packages
Preamble
We can wrap our code in Python modules within our catkin work space to share with other packages in our ROS environment. Why would we do this? It can be great for code re-usability and wrapping commonly used functions and classes. This can dramatically cut down on repetition of code across your ROS packages.
How To
I’m going to start with a fresh catkin work space but feel free to adapt to suit your environment, package naming and file naming conventions.
mkdir -p catkin_ws/src
cd catkin_ws
catkin_make
cd src
catkin_create_pkg my_ros_package rospy
cd my_ros_package
touch setup.py
mkdir -p src/my_ros_package
touch src/my_ros_package/__init__.py
touch src/my_ros_package/my_python_functions.py
If you are adding a Python module to an already existing package you just need to create the setup.py, __init__.py, my_python_functions.py and the sub-directory with the ROS package src directory.
We are trying to end up with a folder structure that looks like this.
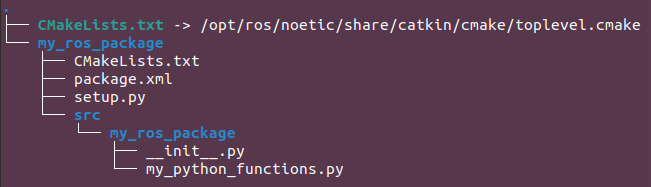
Let’s add a simple tester function.
nano src/my_ros_package/my_python_functions.py
We’ll just use a simple function that adds two numbers together and returns the result.
#!/usr/bin/env python3
def adder(x, y):
out = x + y
return out
Now we need to add some boiler plate code to the setup.py
nano setup.py
Ensure that the strings inside the packages list match the name of the sub-directory inside your ROS packages src directory (multiple entries are allowed). Also be sure that the content of the package_dir dictionary match the path in which your module resides within the ROS package.
from distutils.core import setup
from catkin_pkg.python_setup import generate_distutils_setup
a = generate_distutils_setup(
packages=['my_ros_package'],
package_dir={'': 'src'}
)
setup(**a)
Uncomment out the catkin_python_setup() on line 21 of the boilerplate CMakeLists.txt inside the my_ros_package directory.
## Uncomment this if the package has a setup.py. This macro ensures
## modules and global scripts declared therein get installed
## See http://ros.org/doc/api/catkin/html/user_guide/setup_dot_py.html
catkin_python_setup()
In order to make the module available we need to now make our catkin environment.
cd ..
catkin_make
We can test the availability of the Python module directly in the command line, but the usage would be similar for if you were calling the module in another node.
. ./devel/setup.bash
python3
from my_ros_package.my_python_functions import adder
adder(1, 4)
5
More Practical Applications
- Exposing common functions
- Exposing wrapper classes, useful for registering custom message publishers and having a publish method
- Write out/ read in from a none ROS file type
References
Hey you!
Found this useful or interesting?
Consider donating to support.
Any question, comments, corrections or suggestions?
Reach out on the social links below through the buttons.